Steps to Create a Custom User Model in Django
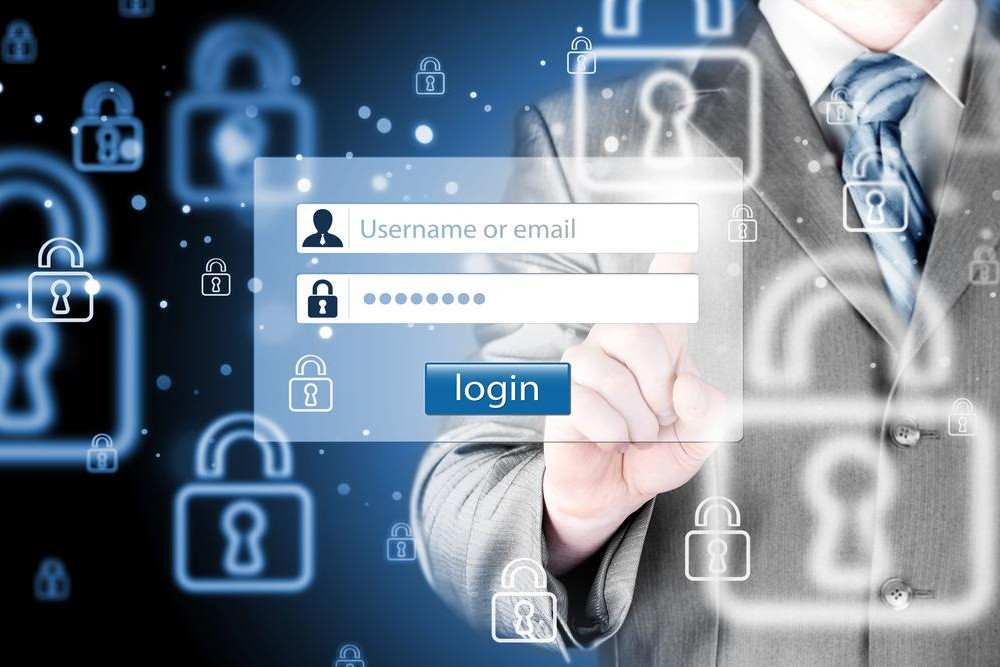
Have you ever wondered why most Python developers prefer Django over other frameworks?
One of the key reasons is that Django offers an easy and flexible way to change or extend the functionality of the default user model to meet complex project requirements.
The default Django User model comes with predefined fields such as username, email, and password, which work well for many web applications.
But what do you do if you need to add extra fields to store additional user information, such as phone numbers, user roles, or profile images? This is where the role of the custom user model comes in.
Before turning to the steps for creating a Django custom user model, let’s understand two base classes of Django for extending the default User model: AbstractBaseUser and AbstractUser.
AbstractBaseUser
The most flexible option provides only the core authentication features, meaning you must clearly define fields like email, username, is_staff, and is_active.
When to Use AbstractBaseUser?
- When you want full control over the user model.
- When you want to remove the username field and use email-based authentication.
- When your application has custom authentication needs (e.g., log in with a phone number).
AbstractUser
This is a pre-built user model that extends Django’s default User model while allowing customization. It includes all the fields from Django’s default User model (username, email, first_name, last_name, etc.), so you don’t have to define them manually.
When to Use AbstractUser?
- When you want to keep Django’s built-in authentication system.
- When you only need minor modifications, such as adding extra fields like phone_number or profile_picture.
- When you want to avoid the extra complexity of AbstractBaseUser.
Now, let’s dive into the steps for creating a custom user model in Django.
Step 1: Create a New Django App (if not already created)
If you don’t already have an app, create one:
python manage.py startapp accounts
Then, add “accounts” to INSTALLED_APPS in settings.py.
Step 2: Choose Between AbstractUser and AbstractBaseUser
- Use AbstractUser if you need minor modifications (e.g., adding fields).
- Use AbstractBaseUser if you need complete customization (e.g., email-based authentication).
Step 3: Create the Custom User Model
Option 1: Extending AbstractUser (Easier Approach)
This keeps the existing Django user model but allows adding custom fields.
from django.contrib.auth.models import AbstractUser
from django.db import models
class CustomUser(AbstractUser):
phone_number = models.CharField(max_length=15, unique=True, blank=True, null=True)
def __str__(self):
return self.username # or return self.email if using email-based login
Option 2: Extending AbstractBaseUser (Advanced Approach)
This requires defining authentication behavior manually.
from django.contrib.auth.models import AbstractBaseUser, BaseUserManager, PermissionsMixin
from django.db import models
class CustomUserManager(BaseUserManager):
def create_user(self, email, password=None, **extra_fields):
if not email:
raise ValueError(“Email is required”)
email = self.normalize_email(email)
user = self.model(email=email, **extra_fields)
user.set_password(password)
user.save(using=self._db)
return user
def create_superuser(self, email, password=None, **extra_fields):
extra_fields.setdefault(‘is_staff’, True)
extra_fields.setdefault(‘is_superuser’, True)
return self.create_user(email, password, **extra_fields)
class CustomUser(AbstractBaseUser, PermissionsMixin):
email = models.EmailField(unique=True)
first_name = models.CharField(max_length=30)
last_name = models.CharField(max_length=30)
is_active = models.BooleanField(default=True)
is_staff = models.BooleanField(default=False)
objects = CustomUserManager()
USERNAME_FIELD = ’email’
REQUIRED_FIELDS = [‘first_name’, ‘last_name’]
def __str__(self):
return self.email
Step 4: Update settings.py
To make Django use this model instead of the default User model, add the following line in
AUTH_USER_MODEL = ‘accounts.CustomUser’
Step 5: Create and Apply Migrations
Run the following commands:
python manage.py makemigrations accounts
python manage.py migrate
Step 6: Register the Model in admin.py
For AbstractUser:
from django.contrib import admin
from django.contrib.auth.admin import UserAdmin
from .models import CustomUser
class CustomUserAdmin(UserAdmin):
fieldsets = UserAdmin.fieldsets + (
(‘Additional Info’, {‘fields’: (‘phone_number’,)}),
)
admin.site.register(CustomUser, CustomUserAdmin)
For AbstractBaseUser:
from django.contrib import admin
from django.contrib.auth.admin import UserAdmin
from .models import CustomUser
class CustomUserAdmin(UserAdmin):
list_display = (’email’, ‘first_name’, ‘last_name’, ‘is_staff’)
search_fields = (’email’, ‘first_name’, ‘last_name’)
ordering = (’email’,)
admin.site.register(CustomUser, CustomUserAdmin)
Step 7: Create a Superuser
Run the command to create an admin user:
python manage.py createsuperuser
For AbstractUser, it will ask for a username.
For AbstractBaseUser, it will ask for an email instead.
Step 8: Test Login & Authentication
Start the server:
python manage.py runserver
By following these steps, you can create a fully functional custom user model in Django.
Are you interested to learn Python Course in Calicut, Kerala? Enroll in our Python Django and Python Django Angular Full Stack Programs to build a career in the tech industry.
Recent Posts
Data Science in 2025: 5 Top Python Libraries
Data Science in 2025: 5 Top Python Libraries Python stands as one of the rapidly-growing coding languages in the world...
Read MoreWhat to Do After Plus Two or Graduation? Learn Python
What’s Next After Plus Two or Graduation? Why Python Might Be the Right Direction Just completed your Plus Two or...
Read MoreTechno Space Academy.
Techno Space Academy greetings. Take charge, grow, and learn. We are more than just a Techno Space Academy; we are...
Read MoreThe Future of Python: Why It’s the Smartest Career Move in 2025
Are you planning to build a successful career in the tech world? If yes, then learning Python in 2025 is...
Read More